4 Textbox (Textbox1, Textbox2, Textbox3, Textbox4)
1 Button (Name: Button1, Text: Set Cursor Position and Log me In"
2 Timers (Timer1 (Interval: 100, Enabled: True), Timer2 (Interval: 10000, Enabled: False)
2 Labels (Name: Label1 (Text: X), Label2 (Text: Y))
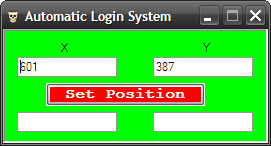
Codes:
Public Class Form1
Public Declare Sub SetCursorPos Lib "user32" (ByVal X As Integer, ByVal Y As Integer)
Public Declare Sub mouse_event Lib "user32" (ByVal dwFlags As Integer, ByVal dx As Integer, ByVal dy As Integer, ByVal cButtons As Integer, ByVal dwExtraInfo As Integer)
Public Const MOUSEEVENTF_LEFTDOWN = &H2
Public Const MOUSEEVENTF_LEFTUP = &H4
Public Const MOUSEEVENTF_RIGHTDOWN = &H8
Public Const MOUSEEVENTF_RIGHTUP = &H10
Private Sub Timer1_Tick(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Timer1.Tick
TextBox1.Text = Cursor.Position.X
TextBox2.Text = Cursor.Position.Y
End Sub
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
Dim first As Integer = TextBox3.Text
Dim second As Integer = TextBox4.Text
SetCursorPos(first, second)
mouse_event(MOUSEEVENTF_LEFTDOWN, 0, 0, 0, 0)
mouse_event(MOUSEEVENTF_LEFTUP, 0, 0, 0, 0)
Timer2.Enabled = True
End Sub
Private Sub Timer2_Tick(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Timer2.Tick
SendKeys.Send("YOUR YOUTUBE USERNAME")
SendKeys.Send("{TAB}")
SendKeys.Send("YOUR YOUTUBE PASSWORD")
SendKeys.Send("{ENTER}")
Timer2.Enabled = False
End Sub
End Class
_________________________________________________________________________
Here is the video tutorial: